单例模式:确保某一个类只有一个实例,并提供全局访问该实例的方法。
uml图如下:
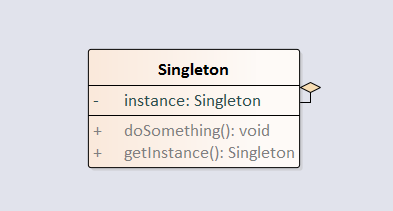
1.饿汉式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.notejava.singleton.hungry;
public class Singleton { private static Singleton instance = new Singleton();
private Singleton() {}
public static Singleton getInstance() { return instance; } }
|
2.懒汉式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.notejava.singleton.lazy;
public class Singleton { private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
|
3.线程安全的懒汉式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.notejava.singleton.secure;
public class Singleton { private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
|
4.双重检测懒汉式(DCL)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.notejava.singleton.dcl;
public class Singleton { private volatile static Singleton instance;
private Singleton() {}
public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } }
|
5.内部静态类式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| package com.notejava.singleton.holder;
public class Singleton { private Singleton() {}
public static Singleton getInstance() { return SingletonHolder.instance; }
private static class SingletonHolder { private static final Singleton instance = new Singleton(); } }
|
6.容器式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.notejava.singleton.manager;
import java.util.HashMap; import java.util.Map;
public class SingleManager { private static Map instanceMap = new HashMap();
private SingleManager() {}
public static void register(String key, Object instance) { if (!instanceMap.containsKey(key)) { instanceMap.put(key, instance); } }
public static Object get(String key) { return instanceMap.get(key); } }
|