简单工厂模式:专门定义一个类来负责创建其他类的实例,被创建的实例通常都具有共同的父类。
例子如下:
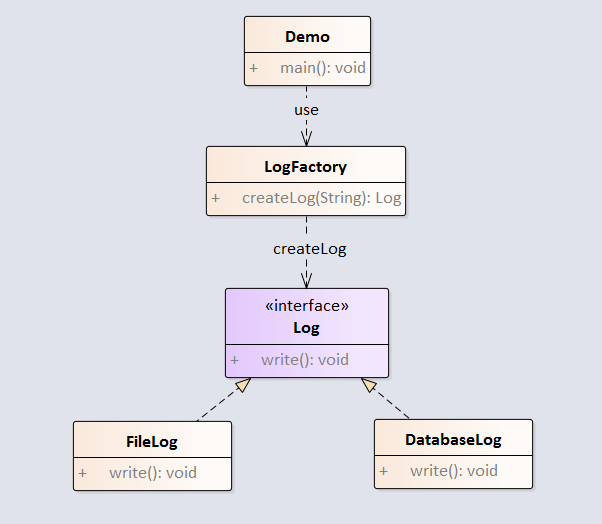
代码实现如下:
1.定义Log接口
1 2 3 4 5 6 7 8
| package com.notejava.factory.simple;
public interface Log { void write(); }
|
2.定义FileLog类
1 2 3 4 5 6 7 8 9 10
| package com.notejava.factory.simple;
public class FileLog implements Log { public void write() { System.out.println("write to file"); } }
|
3.定义DatabaseLog类
1 2 3 4 5 6 7 8 9 10
| package com.notejava.factory.simple;
public class DatabaseLog implements Log { public void write() { System.out.println("write to database"); } }
|
4.定义LogFactory类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.notejava.factory.simple;
public class LogFactory { public Log createLog(String logType) { if ("fileLog".equals(logType)) { return new FileLog(); } if ("databaseLog".equals(logType)) { return new DatabaseLog(); } return null; } }
|
5.定义Demo类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.notejava.factory.simple;
public class Demo { public static void main(String\[\] args) { LogFactory logFactory = new LogFactory(); Log fileLog = logFactory.createLog("fileLog"); if (fileLog != null) fileLog.write(); Log databaseLog = logFactory.createLog("databaseLog"); if (databaseLog != null) databaseLog.write(); } }
|